はじめに
これまでの連載では地図サービスのBing Maps関連のAPI/
Bing APIを利用すると、
今回の内容は、
Bing API
Bing APIでは、
APIによる情報の取得は、
- モバイル向けのWebサイトの検索
- 画像の検索
- 動画の検索
なども可能です。
また、
- キーワードの修正候補や
- 関連キーワード
の取得もできます。
これら以外にも、
Application IDの作成
Bing APIを利用するにあたり、
AppID作成は、
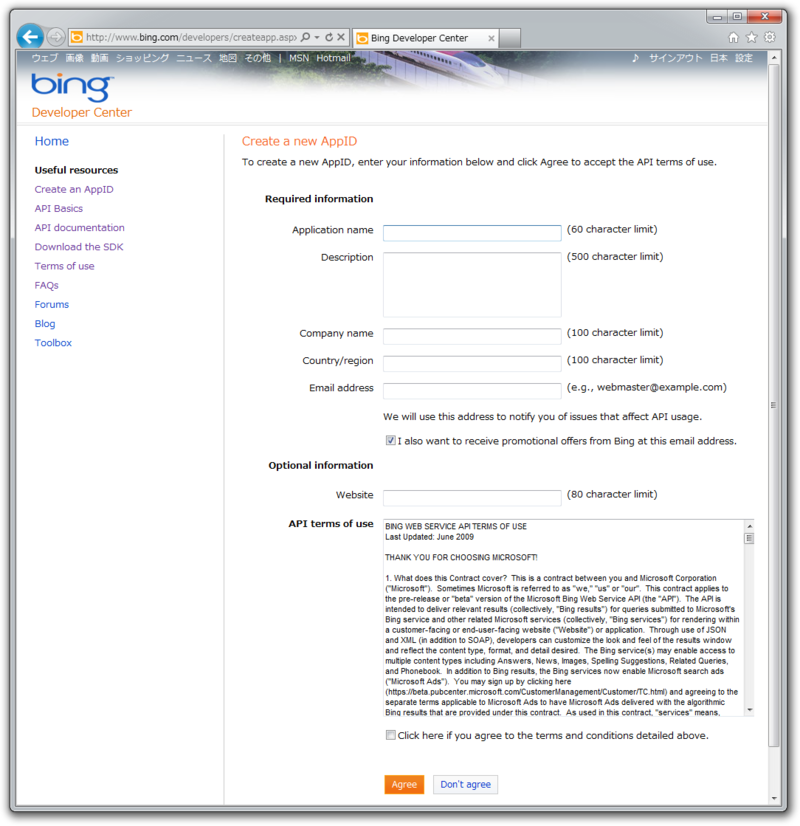
必要な項目を入力し、
Webサイトの検索
それでは、
- http://
api. bing. net/ json. aspx?
AppId=AppId&
Version=2.2 &
Market=ja-JP&
Query=Windows&
Sources=Web&
Web.Count=2&
Web.Offset=0
各パラメーターは次の通りです。
名前 | 説明 |
---|---|
AppID | AppID |
Version | APIのバージョン |
Market | 言語・ |
Query | 検索クエリー |
Sources | 検索対象 Web, Image, Video, RelatedSearch, Spellなど |
Web. |
取得する件数 |
Web. |
取得する位置 |
このほかにもパラメーターはいくつか用意されていますが、
URLにアクセスすると次のようなJSON形式の結果が得られます。簡単ですね。内容は直感でわかるのではないかと思います。ちなみにURL内のjson.
{
"SearchResponse": {
"Query": {
"SearchTerms": "windows"
},
"Version": "2.2",
"Web": {
"Offset": 0,
"Results": [
{
"CacheUrl": "http://cc.bingj.com/cache.aspx?q=windows&d=1&mkt=ja-JP&w=1",
"Description": "Windows 製品とテクノロジの公式ホーム ページです。 (略)",
"DisplayUrl": "www.microsoft.com/japan/windows/default.mspx",
"Title": "Microsoft Windows ホーム ページ",
"Url": "http://www.microsoft.com/japan/windows/default.mspx"
},
{
"CacheUrl": "http://cc.bingj.com/cache.aspx?q=windows&d=5044954658701345&mkt=ja-JP&w=69e7b6a,49d6665a",
"DateTime": "2011-03-05T08:36:00Z",
"Description": "Windows 製品公式ホーム ページです。 (略)",
"DisplayUrl": "www.microsoft.com/japan/windows/default.asp",
"Title": "Windows ホーム: Windows 製品情報、機能比較 ...",
"Url": "http://www.microsoft.com/japan/windows/default.aspx"
}
],
"Total": 414000000
}
}
}
SourcesにWebを指定した場合、
パラメーターに誤りなどがあり、
{
"SearchResponse": {
"Errors": [
{
"Code": 1002,
"HelpUrl": "http://msdn.microsoft.com/en-us/library/dd251042.aspx",
"Message": "Parameter has invalid value.",
"Parameter": "SearchRequest.Web.Count",
"Value": "100"
}
],
"Query": {
"SearchTerms": "windows"
},
"Version": "2.2"
}
}
コードの記述
次は、
<!DOCTYPE html>
<html lang="ja">
<head>
<meta charset="utf-8" />
<title>Bing API Sample</title>
<link rel="stylesheet" href="default.css" type="text/css" />
<!-- jQuery ライブラリーの参照 -->
<script src="http://ajax.aspnetcdn.com/ajax/jquery/jquery-1.5.1.js" type="text/javascript"></script>
<!-- Bing API を利用する JavaScript ファイルの参照 -->
<script src="./web.js" type="text/javascript" charset="utf-8"></script>
</head>
<body>
<!-- 検索ボックス -->
<div id="box"><input type="text" id="query" /><input type="submit" id="search" value="" /></div>
<div style="clear: left;">
<!-- ここに検索の件数を表示する -->
<div id="summary"></div>
<!-- ここに検索結果のWebサイト情報を表示する -->
<ul id="list"></ul>
</div>
</body>
</html>
body, input {
font-family: Meiryo;
}
#box {
border: 1px solid #acbabd;
float: left;
height: 24px;
padding: 2px;
}
#query {
border-width: 0;
float: left;
font-size: medium;
outline-style: none;
width: 175px;
height: 22px;
}
#search {
background-image: url("http://www.bing.com/siteowner/s/siteowner/Spyglass_24x24.gif");
border-width: 0;
cursor: pointer;
float: left;
height: 24px;
width: 24px;
}
#summary {
padding-top: 1em;
color: Gray;
}
#list {
padding: 1em;
}
li {
padding-bottom: 0.5em;
}
.desc {
font-size: small;
}
.dispUrl {
font-size: small;
color: Green;
}
.crawlDate {
font-size: small;
color: Gray;
}
.cache {
font-size: small;
}
$(function () {
// AppId
var appId = "AppID";
// 特殊文字を置換する関数
var esc = function (str) {
if (!str) {
return "";
} else {
return str.replace(/&/g, "&").replace(/\</g, "<").replace(/\>/g, ">");
}
}
// (ここにコードを追記します)
});
コード中のAppIDは、
Bing APIの呼び出し
JavaScriptファイルにコードを追記していきましょう。検索ボックスのボタンをクリックしたときのAPIを呼び出す処理を追加します。コードは次のようになります。
$("#search").click(function() {
var q = $("#query").val();
if (!q) {
// クエリーの入力がない場合は Bing へ移動
location.href = "http://www.bing.com/"
return;
}
// Bing API の非同期呼び出し
$.ajax({
type: "GET",
url: "http://api.bing.net/json.aspx",
dataType: "jsonp",
jsonp: "JsonCallback",
data: { // Bing API のパラメーター
AppId: appId,
Version: "2.2",
Market: "ja-JP",
Query: q,
Sources: "Web",
"Web.Count": 10,
"Web.Offset": 0,
JsonType: "callback" // ← JSONP 形式の結果を指定
},
success: function (data) {
// 非同期呼出しが成功した場合
searchCallback(data);
},
error: function (jqXHR, textStatus, errorThrown) {
// 非同期呼出しに失敗した場合
alert(errorThrown);
}
});
});
ここでのポイントは、
名前 | 説明 |
---|---|
JsonType | レスポンスの種類 以下の3種類から指定
|
JsonCallback | JsonTypeがcallbackの場合、 |
JsonTypeパラメーターにcallbackを指定した場合、
if(typeof userCallback == 'function')
userCallback({"SearchResponse":{/*(省略)*/}});
レスポンスの表示
最後に、
var searchCallback = function (response) {
// 結果がエラーの場合
if (response &&
response.SearchResponse &&
response.SearchResponse.Errors) {
$.each(response.SearchResponse.Errors, function () {
alert("Code: " + this.Code + "\n" +
"Message: " + this.Message + "\n" +
"Parameter: " + this.Parameter + "\n" +
"Value: " + this.Value);
});
return false;
}
var list = $("#list");
list.empty();
var summary = $("#summary");
summary.empty();
if (response &&
response.SearchResponse &&
response.SearchResponse.Web &&
response.SearchResponse.Web.Results) {
// 結果の一覧表示
$.each(response.SearchResponse.Web.Results, function () {
list.append('<li><a href="' + this.Url + '">' + esc(this.Title) + '</a><br />' +
'<span class="desc">' + esc(this.Description) + '</span><br />' +
'<span class="dispUrl">' + esc(this.DisplayUrl) + '</span> ' +
'<span class="crawlDate">' + ((this.DateTime) ? new Date(this.DateTime) : "") + '</span> ' +
'<span class="cache"><a href="' + this.CacheUrl + '">キャッシュ</a></span>' + '</li>');
});
// 件数の表示
var offset = response.SearchResponse.Web.Offset;
summary.html((offset + 1) + "-" + (offset + response.SearchResponse.Web.Results.length) + " 件 " +
"(" + response.SearchResponse.Web.Total + "件中) の検索結果");
} else {
list.append("<li>No results</li>");
}
}
パラメーター不正などのBing APIのエラーの場合は、
以上の実行結果は図2のようになります。正しく動作したでしょうか。
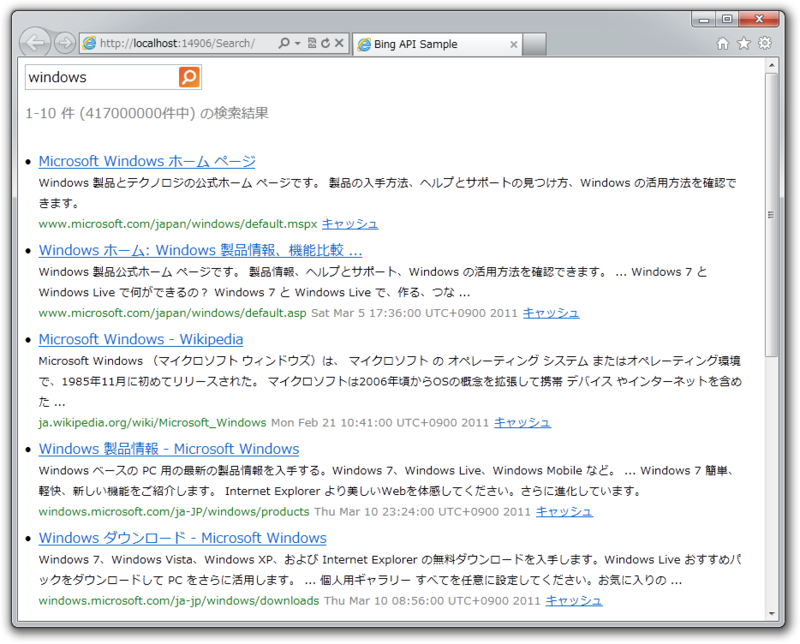
Webサイトの検索に限っては、
今回はここまでです。導入部分しか紹介できていませんので、