はじめに
今回はSilverlightでBing APIを使ってみましょう。Silverlightを使用すれば、
前回までのjQueryでBing APIを利用したときは、
- http://
api. bing. net/ json. aspx?
AppId=AppId&
Version=2.2 &
Market=ja-JP&
Query=Windows&
Sources=Web
jQueryの場合と同様の方法をSilverlightでも記述できますが、
開発環境
はじめに開発環境についてです。Silverlightでの開発は次の環境が必要です。あらかじめインストールを行ってください。
無償のVisual Web Developer Express 2010 ExpressとSilverlight 4 Toolsの組み合わせでも開発できます。Visual Web DeveloperはMicrosoft Web Platform Installer内に含まれています。
またデザイナツールのExpression Blend 4もあるとよいですが、
ライブラリーのインストール
Silverlightアプリケーション開発には必須ではありませんが、
Bing APIサービスの参照
それでは、
プロジェクトの作成
まず、
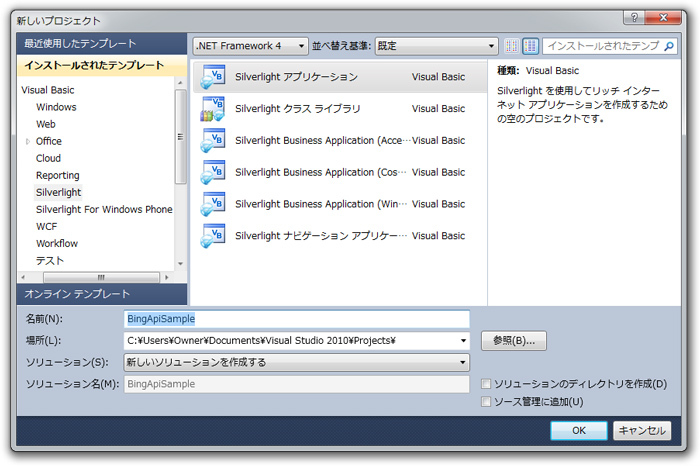
OKボタンをクリックすると、
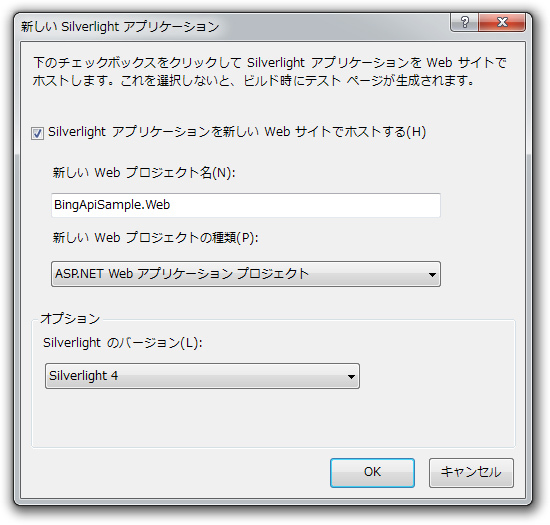
以上で新しい Silverlight アプリケーション プロジェクトと、
最初にインストールしたライブラリーも使用できるようプロジェクトに参照を追加しておきましょう。Visual Studioプロジェクト メニューの参照の追加から、
- System.
CoreEx - System.
Observable - System.
Reactive
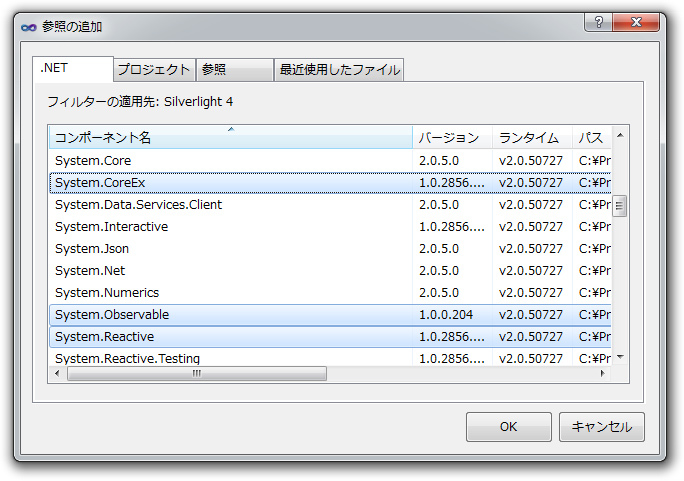
サービス参照の追加
SOAPによるWebサービスの利用は、
プロジェクト メニューからサービス参照の追加を選択します。開いたウィンドウにあるアドレス欄に以下のURLを入力します。
- http://
api. bing. net/ search. wsdl?AppID=AppID&Version=2. 2
このアドレスがBing APIのサービスを定義しているURLです。AppIDパラメーターにはApplication ID
移動ボタンをクリックするとBing APIで提供されているサービス内容が確認できます
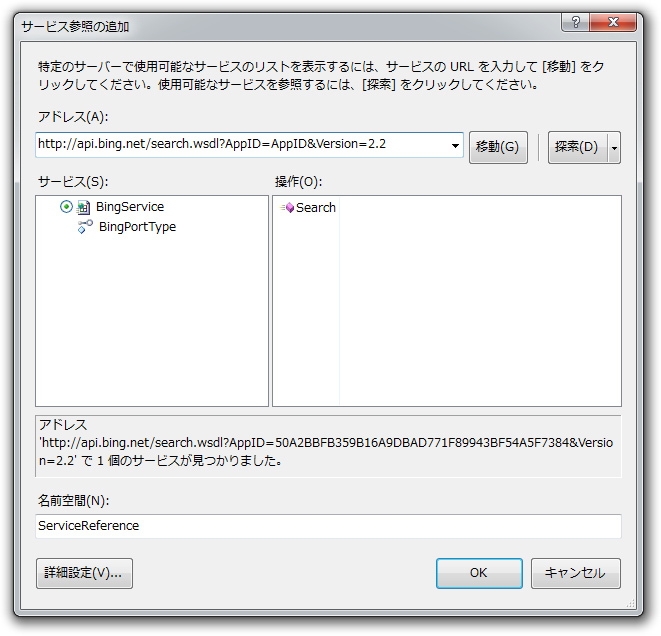
以上で、
Webサイトの検索
次にBing APIを利用してWebサイトを検索します。検索クエリーを入力と、
画面の作成
SilverlightではXAMLと呼ばれるXMLベースの言語によって画面が構成されています。今回は直接XAMLを記述して画面を作成します。ソリューションエクスプローラーからMainPage.
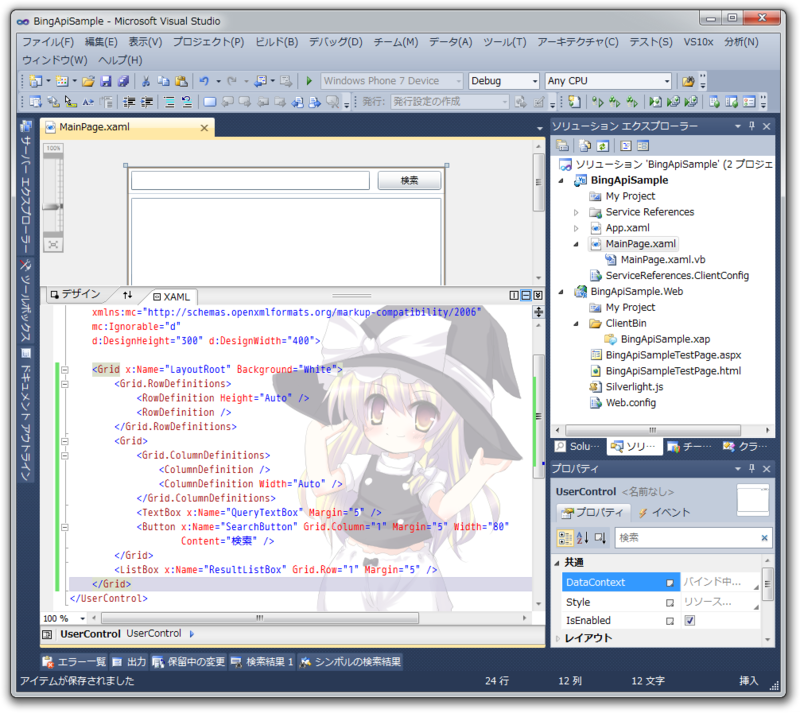
※図では、
ここに、
<Grid x:Name="LayoutRoot" Background="White">
<Grid.RowDefinitions>
<RowDefinition Height="Auto" />
<RowDefinition />
</Grid.RowDefinitions>
<Grid>
<Grid.ColumnDefinitions>
<ColumnDefinition />
<ColumnDefinition Width="Auto" />
</Grid.ColumnDefinitions>
<TextBox x:Name="QueryTextBox" Margin="5" />
<Button x:Name="SearchButton" Grid.Column="1" Margin="5" Width="80"
Content="検索" />
</Grid>
<ListBox x:Name="ResultListBox" Grid.Row="1" Margin="5" />
</Grid>
この後にも編集しますが、
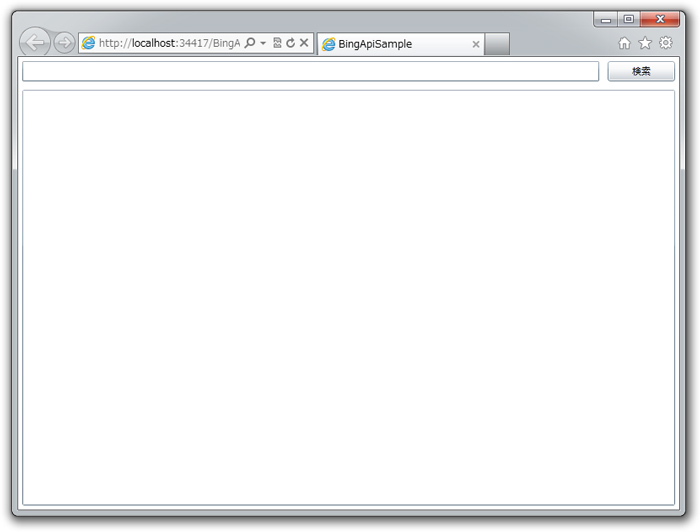
コードの記述
続いてAPIの呼び出しなどを行うコードを記述します。ソリューションエクスプローラーからMainPage.
Imports BingApiSample.ServiceReference
次に、
Private Sub Search(ByVal query As String)
If query = "" Then
' クエリーが指定されていない場合は処理を抜ける
Exit Sub
End If
' (ここに Bing API の呼び出し処理を記述)
End Sub
ボタンをクリックしたとき、
Public Sub New()
InitializeComponent()
AddHandler SearchButton.Click, Sub() Search(QueryTextBox.Text)
End Sub
検索処理の手順は次のように行います。必ずしもこうしなければならないというものではありません。
- BintPortTypeClientオブジェクトの生成
- 検索が完了したときの処理を定義
(リストボックスにレスポンス内容を表示) - 検索リクエストの作成
- 検索API呼出し
まずBintPortTypeClientオブジェクトを生成します。自動生成されたクラスでしたね。
Dim client = New BingPortTypeClient
APIの呼出しは非同期で行います。レスポンスを受信
' API 呼出し完了したときの処理(リストボックスに結果を表示)
Observable.FromEvent(Of SearchCompletedEventArgs)(client, "SearchCompleted") _
.Take(1) _
.Subscribe(
Sub(e)
If e.EventArgs.Error Is Nothing Then
Dim result = e.EventArgs.Result
ResultListBox.ItemsSource = If(result.Web IsNot Nothing, result.Web.Results, Nothing)
End If
End Sub)
リクエストの作成は次のようになります。AppIDや検索クエリーなど検索の全般的な設定を指定は、
' SearchRequest と WebRequest の作成
Dim request = New SearchRequest With {
.AppId = "AppID",
.Market = "ja-JP",
.Query = query,
.Sources = {SourceType.Web},
.Web = New WebRequest With {
.Offset = 0,
.Count = 10}
}
最後に、
client.SearchAsync(request)
ここまでを実行してみましょう。テキストボックスにクエリーを入力し検索ボタンをクリックすると図7のようになるかと思います。
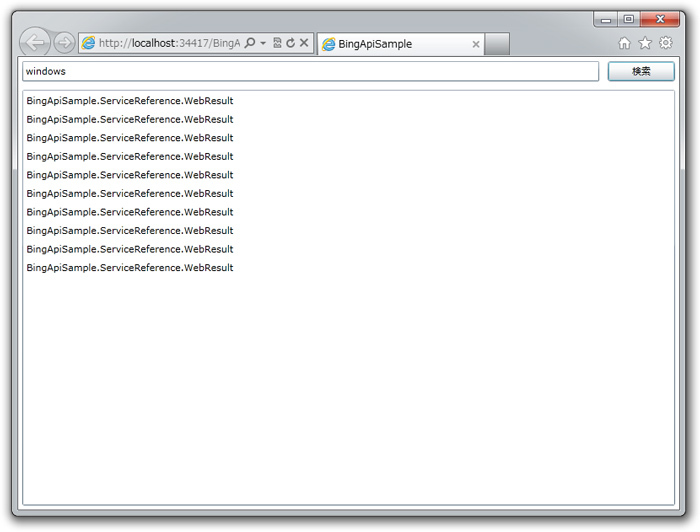
リストボックスにWebサイトの検索結果
デザインの編集
リストボックスに表示される項目
<ListBox x:Name="ResultListBox" Grid.Row="1" Margin="5"
ScrollViewer.HorizontalScrollBarVisibility="Disabled">
<ListBox.ItemTemplate>
<DataTemplate>
<StackPanel Margin="0 0 0 10">
<HyperlinkButton NavigateUri="{Binding Url}" TargetName="_blank">
<TextBlock Text="{Binding Title}" />
</HyperlinkButton>
<TextBlock Text="{Binding Description}" TextWrapping="Wrap" />
</StackPanel>
</DataTemplate>
</ListBox.ItemTemplate>
</ListBox>
検索結果のデータの表示方法は、
もう一度、
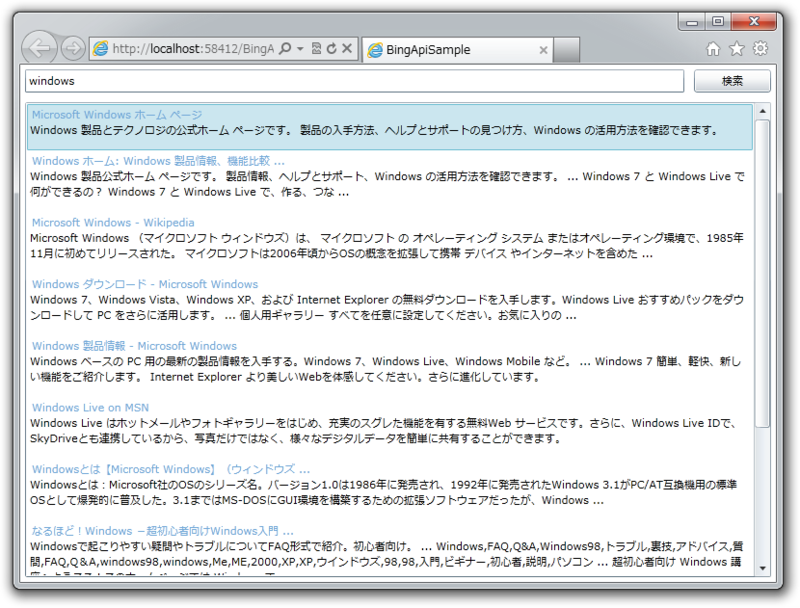
以上で、
Webサイト検索結果のプロパティ
使用したWebResultオブジェクトのプロパティは、
名前 | 説明 |
---|---|
DateTime | 最終更新日またはクロールした時間 例: 2011-03-30T07:20:00Z |
Description | 説明 |
DeepLinks | Webサイトに含まれるリンク 各アイテムはTitleとUrlプロパティを持っています。 |
DisplayUrl | 表示用のURL |
SearchTags | 検索用タグ <meta>タグのname属性が |
Title | Webサイトのタイトル |
Url | WebサイトのURL |
CacheUrl | WebサイトのキャッシュのURL |
ただし、
<ListBox x:Name="ResultListBox" Grid.Row="1" Margin="5"
ScrollViewer.HorizontalScrollBarVisibility="Disabled">
<ListBox.ItemTemplate>
<DataTemplate>
<StackPanel Margin="0 0 0 10">
<HyperlinkButton NavigateUri="{Binding Url}" TargetName="_blank">
<TextBlock Text="{Binding Title}" />
</HyperlinkButton>
<TextBlock Text="{Binding Description}" TextWrapping="Wrap" />
<TextBlock Text="{Binding DateTime}" />
<TextBlock Text="{Binding DisplayUrl}" />
<TextBlock Text="{Binding CacheUrl}" />
<ListBox Margin="10 0 0 0" ItemsSource="{Binding DeepLinks}">
<ListBox.ItemTemplate>
<DataTemplate>
<HyperlinkButton NavigateUri="{Binding Url}" TargetName="_blank" Content="{Binding Title}" />
</DataTemplate>
</ListBox.ItemTemplate>
</ListBox>
<ListBox Margin="10 0 0 0" ItemsSource="{Binding SearchTags}">
<ListBox.ItemTemplate>
<DataTemplate>
<StackPanel Orientation="Horizontal">
<TextBlock Text="{Binding Name}" />
<TextBlock Text="{Binding Value}" Margin="5 0 0 0" />
</StackPanel>
</DataTemplate>
</ListBox.ItemTemplate>
</ListBox>
</StackPanel>
</DataTemplate>
</ListBox.ItemTemplate>
</ListBox>
送受信内容の確認
ここで、
確認方法は簡単です。Fiddler2を起動後、
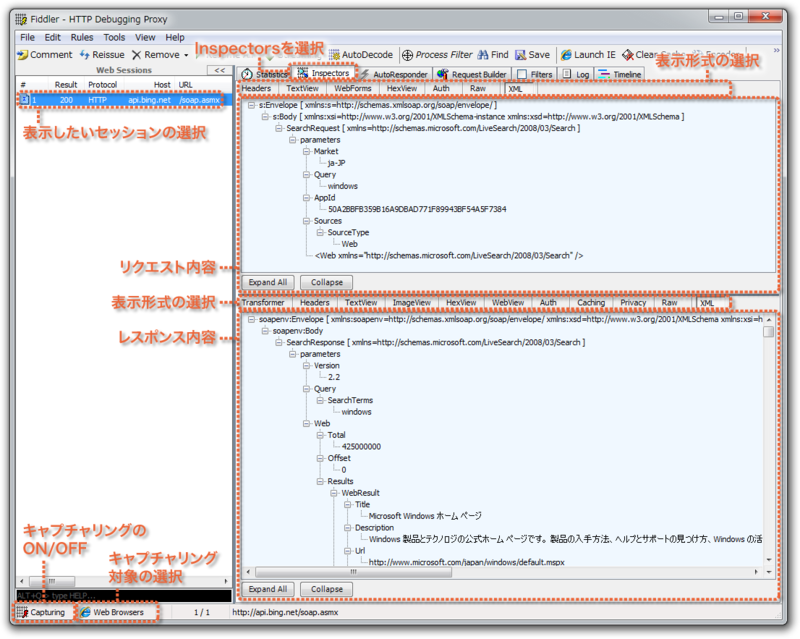
Visual Studioを使用したため実際の通信処理の部分は隠ぺいされた形になっていましたが、
画像の検索
作成したSilverlightアプリケーションのコードを変更して、
まず、
' API 呼出し完了したときの処理(リストボックスに結果を表示)
Observable.FromEvent(Of SearchCompletedEventArgs)(client, "SearchCompleted") _
.Take(1) _
.Subscribe(
Sub(e)
If e.EventArgs.Error Is Nothing Then
Dim result = e.EventArgs.Result
ResultListBox.ItemsSource = If(result.Image IsNot Nothing, result.Image.Results, Nothing)
End If
End Sub)
リクエストの作成も次のようにImageRequestオブジェクトとSourceType.
' SearchRequest と ImageRequest の作成
Dim request = New SearchRequest With {
.AppId = "AppID",
.Market = "ja-JP",
.Query = query,
.Sources = {SourceType.Image},
.Image = New ImageRequest With {
.Offset = 0,
.Count = 10}
}
次は、
<ListBox x:Name="ResultListBox" Grid.Row="1" Margin="5"
ScrollViewer.HorizontalScrollBarVisibility="Disabled">
<ListBox.ItemTemplate>
<DataTemplate>
<StackPanel Margin="0 0 0 10">
<HyperlinkButton NavigateUri="{Binding Url}" TargetName="_blank">
<TextBlock Text="{Binding Title}" />
</HyperlinkButton>
<Image Source="{Binding Thumbnail.Url}" Width="{Binding Thumbnail.Width}" Height="{Binding Thumbnail.Height}" HorizontalAlignment="Left" />
</StackPanel>
</DataTemplate>
</ListBox.ItemTemplate>
</ListBox>
画像の検索結果特有のThumbnailオブジェクトを<Image>要素で表示するように変更しました。実行結果は図10のようになります。
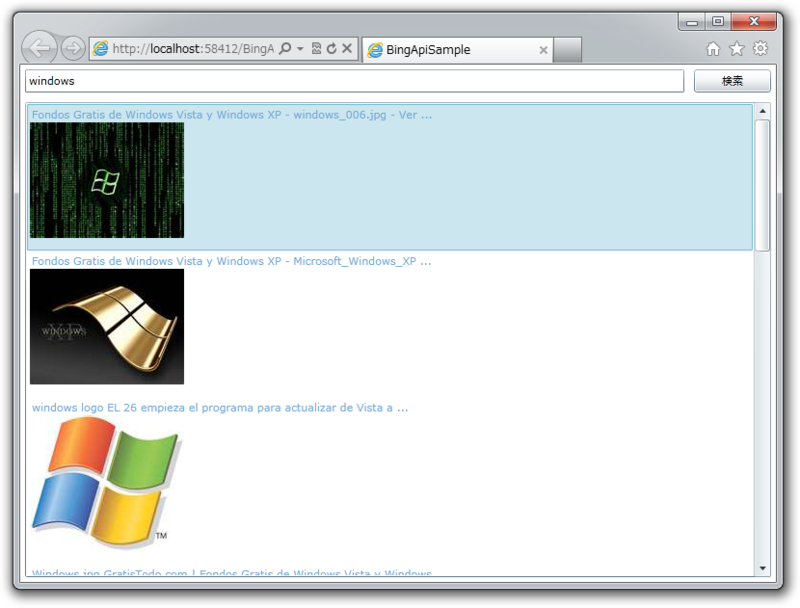
複数の対象を検索
Bing APIでは、
同時に検索するには、
' SearchRequest の作成
Dim request = New SearchRequest With {
.AppId = "AppID",
.Market = "ja-JP",
.Query = query,
.Sources = {SourceType.Web, SourceType.RelatedSearch, SourceType.Image},
.Web = New WebRequest With {
.Offset = 0,
.Count = 10},
.Image = New ImageRequest With {
.Offset = 0,
.Count = 10}
}
関連キーワードのオプションはないため、
次に、
<Grid Grid.Row="1">
<Grid.ColumnDefinitions>
<ColumnDefinition />
<ColumnDefinition />
<ColumnDefinition />
</Grid.ColumnDefinitions>
<!-- 関連キーワード -->
<ListBox x:Name="KeywordResultListBox" Margin="5" ScrollViewer.HorizontalScrollBarVisibility="Disabled">
<ListBox.ItemTemplate>
<DataTemplate>
<HyperlinkButton NavigateUri="{Binding Url}" Content="{Binding Title}" TargetName="_blank" />
</DataTemplate>
</ListBox.ItemTemplate>
</ListBox>
<!-- Webサイト -->
<ListBox x:Name="WebResultListBox" Grid.Column="1" Margin="5" ScrollViewer.HorizontalScrollBarVisibility="Disabled">
<ListBox.ItemTemplate>
<DataTemplate>
<StackPanel Margin="0 0 0 10">
<HyperlinkButton NavigateUri="{Binding Url}" Content="{Binding Title}" TargetName="_blank" />
<TextBlock Text="{Binding Description}" TextWrapping="Wrap" />
</StackPanel>
</DataTemplate>
</ListBox.ItemTemplate>
</ListBox>
<!-- 画像 -->
<ListBox x:Name="ImageResultListBox" Grid.Column="2" Margin="5" ScrollViewer.HorizontalScrollBarVisibility="Disabled">
<ListBox.ItemTemplate>
<DataTemplate>
<StackPanel Margin="0 0 0 10">
<HyperlinkButton NavigateUri="{Binding Url}" Content="{Binding Title}" TargetName="_blank" />
<Image Source="{Binding Thumbnail.Url}" Width="{Binding Thumbnail.Width}" Height="{Binding Thumbnail.Height}" HorizontalAlignment="Left" />
</StackPanel>
</DataTemplate>
</ListBox.ItemTemplate>
</ListBox>
</Grid>
MainPage.
' API 呼出し完了したときの処理(リストボックスに結果を表示)
Observable.FromEvent(Of SearchCompletedEventArgs)(client, "SearchCompleted") _
.Take(1) _
.Subscribe(
Sub(e)
If e.EventArgs.Error Is Nothing Then
Dim result = e.EventArgs.Result
KeywordResultListBox.ItemsSource = If(result.RelatedSearch IsNot Nothing, result.RelatedSearch.Results, Nothing)
WebResultListBox.ItemsSource = If(result.Web IsNot Nothing, result.Web.Results, Nothing)
ImageResultListBox.ItemsSource = If(result.Image IsNot Nothing, result.Image.Results, Nothing)
End If
End Sub)
実行結果は図11のようになります。
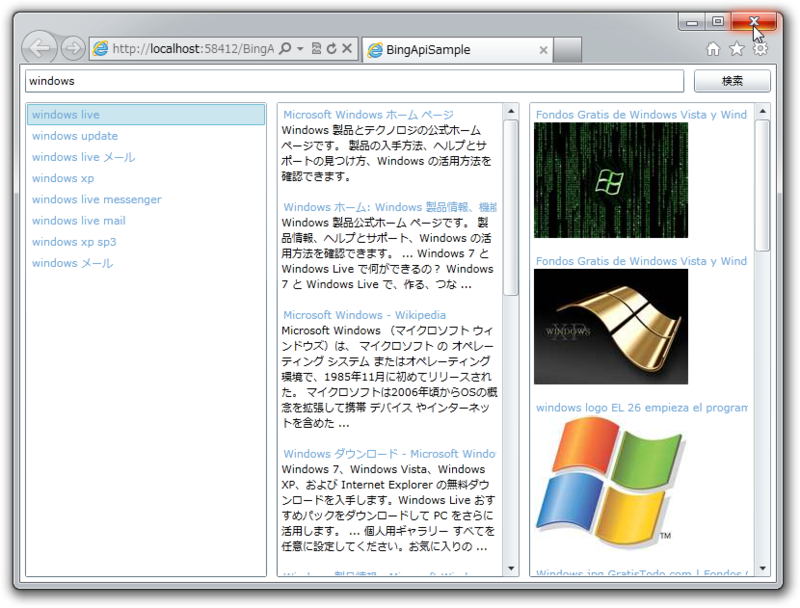
おわりに
今回のアプリケーションを公開する場合は、
- ClientBn/
BingApiSample. xap - BingApiSampleTestPage.
html - Silverlight.
js
Webサーバーによっては、
また、
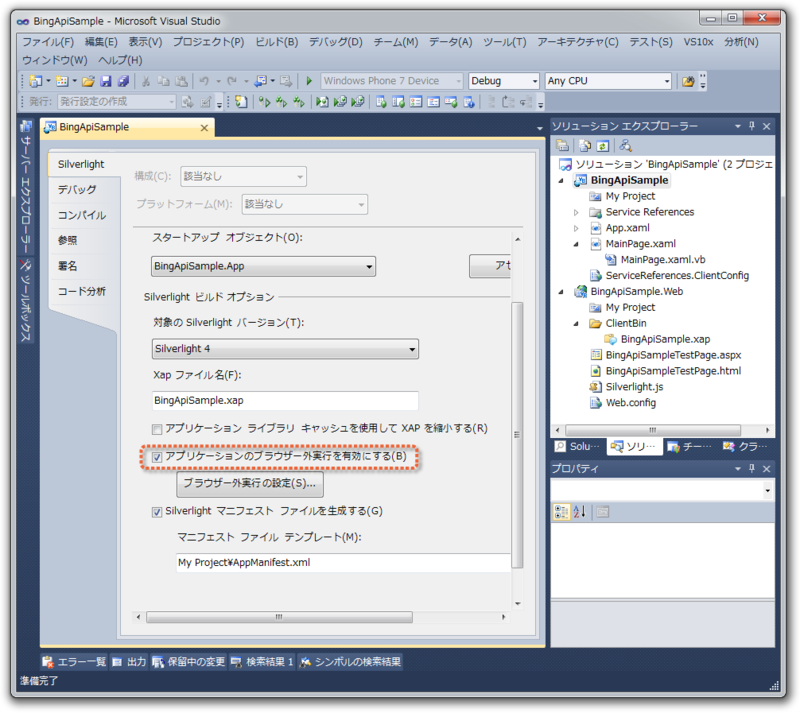
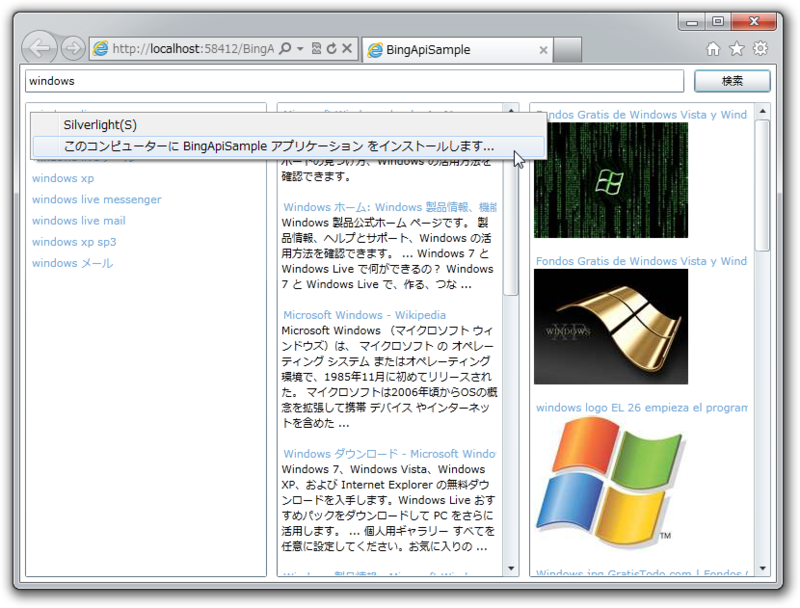
これが冒頭で紹介した、
今回は以上です。いかがでしたでしょうか。次回もSilverlightによるBing APIの利用を紹介する予定です。